Modernize Your JavaScript Grids With Sorting, Grouping, And Filtering
JavaScript grids are important components of many applications. They assist in presenting and visualizing bulk data. Grids organize information in an orderly fashion. They also enable users to perform actions on the data. Functionalities like sorting, grouping, and filtering make grids more powerful. These features improve ease of use. Such functionalities are crucial for dashboards, inventory systems, and analytical tools.
Adding these features to grids has many advantages. It makes the system more convenient. It also improves the structure of data. Users can browse, filter, or analyze complex data sets effortlessly. This boosts productivity and helps in faster decision-making. Enhanced grids are no longer optional; they are mandatory.
There are methods to implement these features step by step. We will demonstrate them in this blog. We’ll use the Sencha Ext JS framework. This framework is one of the best and offers a robust grid. You’ll learn to configure sorting for one or more columns. We’ll also show how to group data on the fly and apply filtering as needed. Each feature will be explained using simple examples.
In this article, we’ll explore ways to improve your grids. The goal is to make them more user-friendly. We’ll first discuss how to make JavaScript grids smarter, faster, and more engaging.
Understanding Basics
A grid is an interactive graphical user interface element. It formats and displays data in grid form on a web application. When implemented, this function organizes and represents large amounts of data. It helps users view, analyze, and interact with data more effectively.
Let’s dive into the main components of a JavaScript grid:
- Rows: Rows are the horizontal arrangement of data in the grid. A row consists of multiple cells aligned on a horizontal axis. Rows hold data in a complete perspective.
- Columns: Columns form the vertical structure of the grid. They represent specific data attributes like “Name,” “Age,” or “Email ID.” Columns contain cells from every row, filled with values for that column.
- Cells: Cells are the smallest units in the grid. A cell is the intersection of a row and a column. It holds a specific data value at that intersection.
Choosing the Right JavaScript Framework
Choosing a JavaScript framework for data grids is a crucial decision. Consider these factors:
- Integration: Ensure the framework integrates seamlessly with your application.
- Features: Look for features like sorting, filtering, grouping, and pagination. These enhance grid functionality.
- Customization: The framework should allow customization of layouts, styles, and appearance.
- Support: Choose a framework with good documentation and an active developer community.
Why Use Ext JS?
Ext JS is ranked among the best for JavaScript grids. It offers over 140 UI components, including a strong and user-friendly grid. Its grid features include grouping, sorting, filtering, and pagination. It handles large datasets efficiently without performance issues.
Ext JS integrates with any web application. It works across different browsers and platforms. It also has extensive documentation and a supportive community.
With Ext JS, your web app will have a responsive, feature-rich, and easy-to-use data grid. It’s a proven tool for effective data management.
Useful JavaScript Data Grid Libraries
Here are some excellent JavaScript libraries:
Sencha Ext JS
Ext JS is a robust framework for mobile and web applications. It’s ideal for data-based apps. It includes over 150 UI components, such as grids, pivot grids, and charts.
Key Features of Sencha Ext JS
- It has over 150 UI elements. These elements are ready to use.
- These elements support advanced grids, pivot grids, and interactive charts.
- Applications developed in Ext JS can run on several platforms.
- Ext JS is suitable for developing data-driven applications. Examples include CRMs or financial applications.
- The framework handles D3.js. This enables the creation of powerful visualizations for data.
- Ext JS is widely used for enterprise-level applications. It guarantees high efficiency and scalability.
AG Grid
- AG Grid is an enterprise-grade JavaScript data grid. It maximizes performance while maintaining flexibility.
- The grid effortlessly handles large volumes of data. It performs tasks like sorting, filtering, grouping, and paging.
- Its appearance can be altered as desired.
- AG Grid employs both client-side and server-side data handling. This adds complexity to web applications.
- It works perfectly with React, Angular, and Vue.
- Every grid comes with detailed documentation and an active developer community.
- The enterprise edition includes features like pivoting and advanced filtering. It ensures a constant flow of information for sensitive applications.
Bryntum Grid
- Bryntum Grid is a modern and easy-to-use grid. It has powerful features and great performance.
- It handles tasks like sorting, filtering, and grouping with ease.
- Users can modify data through in-line editing.
- Bryntum Grid transfers significant amounts of data efficiently. It suits both enterprise and other settings.
- It allows customization. You can build it the way you want to.
- The grid works well with React, Angular, and Vue.
- Features include Excel export, responsive design, and row summaries.
- It provides necessary instructions for a smooth experience.
- Professionals offer assistance, making it a safe option for complex web applications.
How Can I Turbocharge My JavaScript Grids with Sorting?
Controlling data display is crucial in Ext JS. Grids give users control over how data is organized and displayed. Sorting can be configuration-based or programmed. Users can sort data by one column or several at once. This helps them view large datasets in their preferred style.
For example, users can filter flight times by destination or airline.
Steps for Implementing Single-Column Sorting
Specify the View and Extend Grid
First, define the view and extend the grid. Set the title and items.
Ext.define('MyApp.view.Main', {
extend: 'Ext.grid.Grid',
title: 'Reykjavik Flight Departures',
items: [{
docked: 'top',
xtype: 'toolbar',
items: [{
text: 'Sort on destination',
handler: function(button) {
// Sort under program control
button.up('grid').getStore().sort('to');
}
}]
}],
});
A grid titled “Reykjavik Flight Departures” shows this in action. A toolbar includes a button for sorting by destination.
Sort the Data via Configuration
Sort the store to achieve desired results. The store manages the data. Configure the store to sort data by the ‘airline’ property. Data is fetched from Departures.json via AJAX.
store: {
// Sort via store configuration
sorters: [{
property: 'airline'
}],
type: 'store',
autoLoad: true,
fields: [{
name: 'date',
type: 'date',
dateFormat: 'j. M'
}],
proxy: {
type: 'ajax',
url: 'departures.json',
reader: {
rootProperty: 'results'
}
}
}
Define the Columns
Establish the grid’s columns to determine visible fields. Check the source code for more details on defining columns.
Add the Application Code
Start the application and link the main view. This initializes the app and prepares the main view.
Ext.application({
name: 'MyApp',
mainView: 'MyApp.view.Main'
});
This initializes the app and loads the main view.
Multi-Column Sorting
Both airline and destination columns can be sorted together. Multi-column sorting handles complex datasets efficiently. Add more columns to refine sorting as needed. Ext JS also supports multi-column sorting.
Ext JS Grids provide powerful features for sorting and organizing data. You can sort by a single column or multiple columns. This flexibility makes it easier to display data exactly as you need it.
Source Code:
You can play with the code on Fiddle. Next, let’s look at multiple-column sorting. All you have to do to achieve this is add multiple sorters via configuration. Here is an example:
Source Code:
You can find the source code right here.
How Can I Turbocharge My JavaScript Grids with Grouping?
Grouping arranges data into manageable sections. Sencha Ext JS allows easy grouping using the grouped configuration. Follow simple steps to enable grouping in your grid:
Start Here
Specify the View and Title
First, define the view and title of your application. Extend the grid class to specify the properties. For example:
Ext.define('MyApp.view.Main', {
extend: 'Ext.grid.Grid',
title: 'Reykjavik Flight Departures',
});
Set the Grouped Property
To enable column grouping, simply set the grouped property to true:
grouped: true,
Define the Columns
Specify the columns you want to display. You can include various column types, like text, number, or date. You can find everything in the source code, such as:
columns: [
{ text: 'Date', dataIndex: 'date', xtype: 'datecolumn', format: 'j. M' },
{ text: 'Flight No', dataIndex: 'flightNo' },
{ text: 'Destination', dataIndex: 'destination' }
],
Set Up the Data Store
Define the store that will hold the data. In this example, we use an AJAX proxy to load data from an external JSON file:
store: {
type: 'store',
autoLoad: true,
fields: [{ name: 'date', type: 'date', dateFormat: 'j. M' }],
proxy: {
type: 'ajax',
url: 'departures.json',
reader: { rootProperty: 'results' }
}
},
Launch the Application
Finally, wrap everything up in an Ext JS application:
Ext.application({
name: 'MyApp',
mainView: 'MyApp.view.Main'
});
By following these simple steps, you can easily group your data in grids and improve the overall user experience. Grouping helps in organizing and navigating through large datasets, especially when dealing with data like flight departures, sales reports, or employee schedules.
Source Code:
You can find the source code at Fiddle.
How can I Turbocharge my JavaScript Grids with Filtering?
Turbocharging your JavaScript grids with filtering capabilities is a great way to enhance the user experience and streamline data interaction. Using the grid filters plugin, you can implement powerful filtering options, allowing users to easily find the data they need. Here’s a step-by-step guide to implementing filtering in your JavaScript grids:
Start by specifying the app’s view and title: You must create a base structure for your app. This is done by defining the view and title of your app and then extending the Grid component. This provides the fundamental setup needed for your grid.
Enable Grid Filtering: To enable filtering, set gridfilters to true within the plugin section of your grid configuration. This turns on the filtering functionality for the grid.
plugins: {
gridfilters: true
}
Clear Filters Function: You can also create a function to clear applied filters. This is helpful when users want to reset the grid to its initial state. The function will clear any filters applied to the store.
onClearFilters: function() {
this.getStore().clearFilter();
}
Add Columns: Define the columns you want to display in the grid. These should correspond to the data you plan to filter. You can refer to the source code for specific details.
Define Store with AutoLoad: Set up the store, specifying the data fields and enabling the autoLoad option. The store should also be connected to a proxy that loads data via an AJAX request.
store: {
type: 'store',
autoLoad: true,
fields: [{name: 'date', type: 'date', dateFormat: 'j. M'}],
proxy: {
type: 'ajax',
url: 'departures.json',
reader: {rootProperty: 'results'}
}
}
Launch the Application: Finally, initialize the application and specify the main view. This ensures the grid is loaded and ready for interaction.
Ext.application({
name: 'MyApp',
mainView: 'MyApp.view.Main'
});
Source Code:
You can play with the code right here.
Conclusion
Modernizing JavaScript grids with sorting, grouping, and filtering improves the user experience. These features make data interaction and organization easier. Users can manage large datasets more efficiently. This is especially useful for applications like dashboards and inventory systems. Sorting allows users to arrange data by one or more columns.
Grouping organizes data into logical sections. Filtering helps users find the data they need quickly. Using frameworks like Sencha Ext JS, developers can add these features easily. These functionalities make grids more dynamic and user-friendly. They enhance both performance and usability.
FAQs
What are the benefits of modernizing JavaScript grids with sorting, grouping, and filtering?
Modern grids improve the user experience. They provide better data organization and interactivity. Users can quickly find, analyze, and manipulate data. This makes web applications more efficient and user-friendly.
Which JavaScript libraries or frameworks are best for implementing advanced grid features?
Libraries like Ag-Grid, DataTables, Handsontable, and React Table are great options. They offer sorting, grouping, and filtering out of the box. These libraries are customizable and perform well. They integrate seamlessly with frameworks like React, Angular, and Vue.
How can I add sorting functionality to my JavaScript grid?
Most grid libraries include built-in sorting options. For custom solutions, you can use JavaScript’s Array.sort() method. Sort the data and dynamically re-render the grid. You can implement single-column or multi-column sorting as needed.
What is the best way to implement grouping in JavaScript grids?
Grouping organizes rows by shared column values. Libraries like Ag-Grid provide native grouping support. Alternatively, you can pre-process data into nested structures. Render grouped rows with collapsible or expandable sections for better usability.
How do I implement filtering in JavaScript grids to handle large datasets?
For large datasets, use server-side filtering. The grid sends filter criteria to the server, which processes and returns the data. For smaller datasets, client-side filtering is sufficient. Use JavaScript’s filter() method or library-provided tools for quick implementation.
Register yourself today at Sencha Ext JS – Benefit from the amazing JS grid solutions.
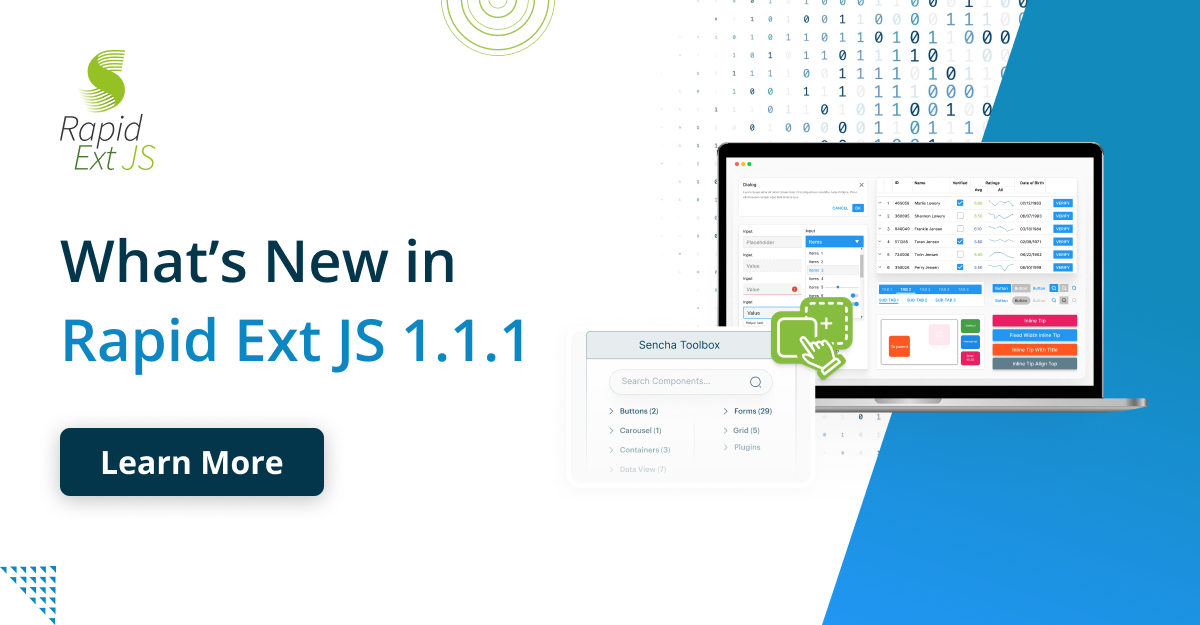
The Sencha team is excited to announce the latest Sencha Rapid Ext JS 1.1.1 release…
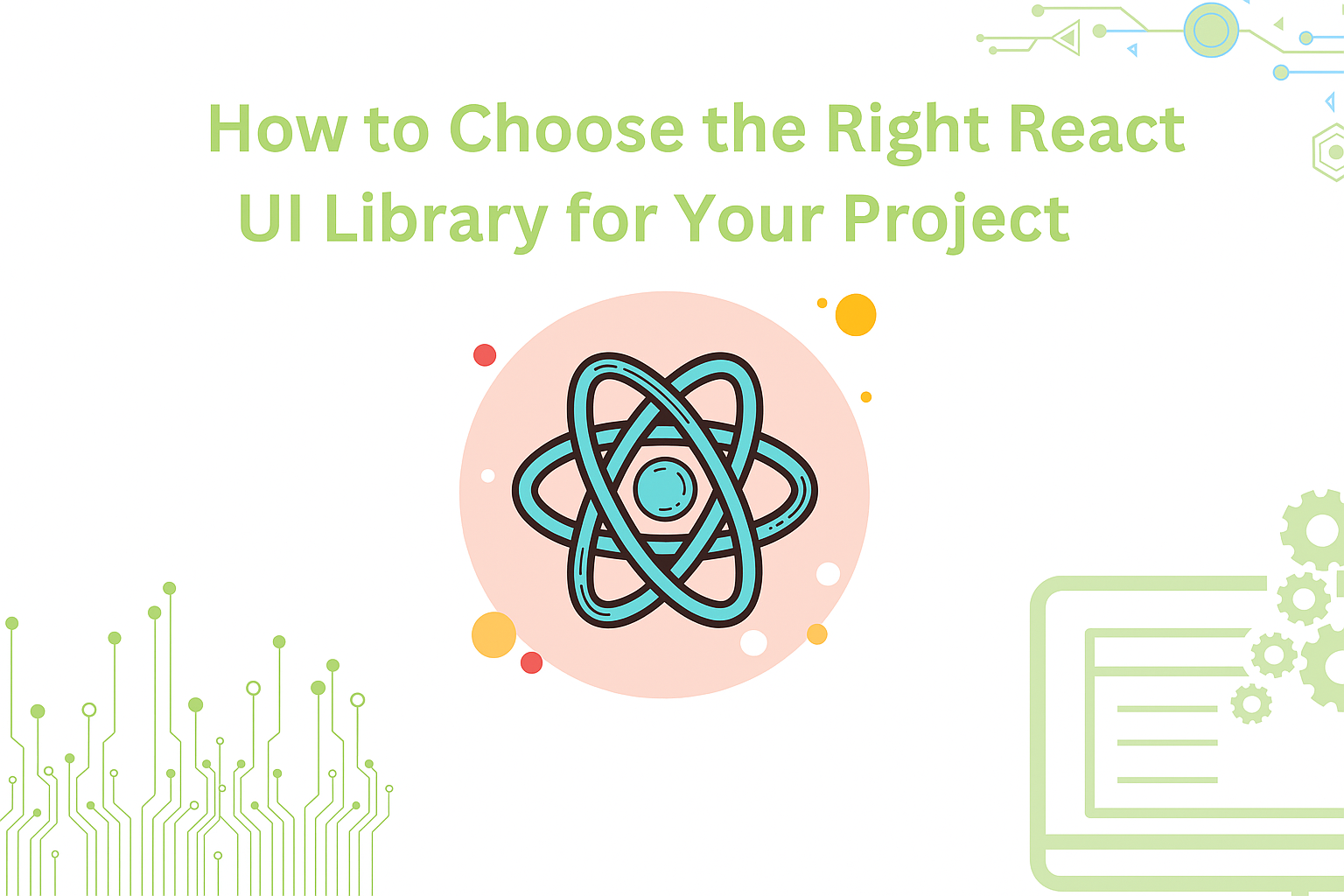
React is perhaps the most widely used web app-building framework right now. Many developers also…
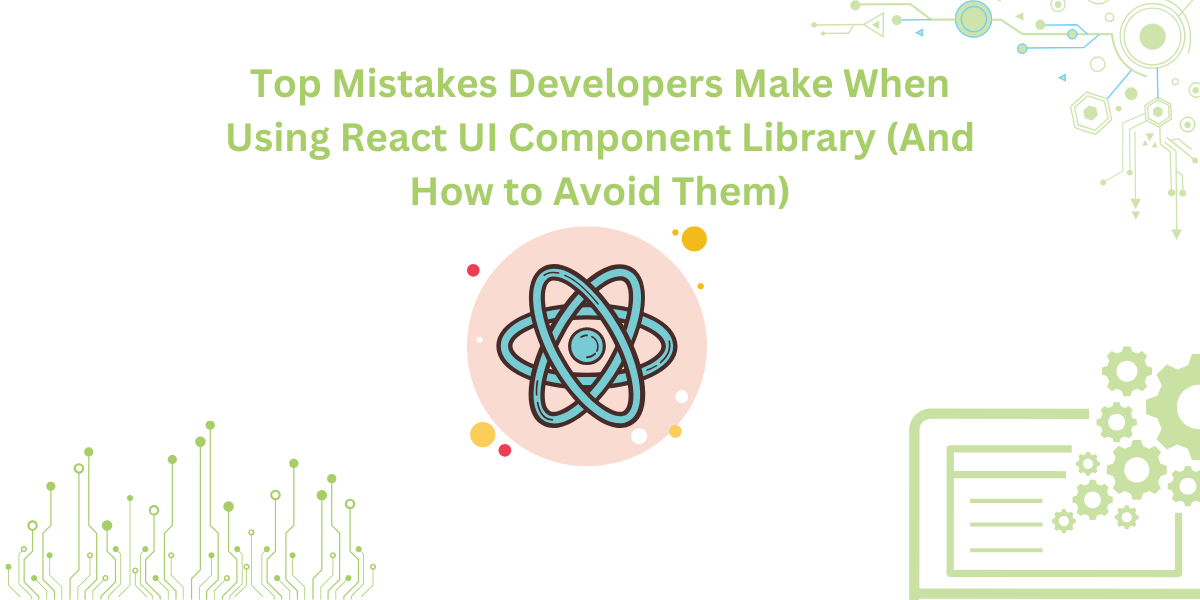
React’s everywhere. If you’ve built a web app lately, chances are you’ve already used it.…